Wrap functions with typeshave to prevent functions doing garbage-in/garbage-out.
Validate nested structures like a boss!
PHP BASICS
function foo($foo, $bar){
TypeShave::check( func_get_args(), (object)array(
"foo" => (object)array( "type" => "string" ),
"bar" => (object(array( "type" => "integer" )
));
// do stuff with valid data
}
oh heck, we can just write jsonschema as well:
function foo($foo, $bar){
TypeShave::check( func_get_args(), '{
"foo": { "type": "string" },
"bar": { "type": "integer" }
}');
// do stuff with valid data
}
TYPESHAVE
uses the established jsonschema validation-format, which allows extensive nested data-validation.
see the composer module here or the github repo here
NODEJS BASICS
COFFEESCRIPT JAVASCRIPT
============ ==========
foo = typesafe var foo = typesafe({
foo: { type: "string" } foo: { type: "string" }
bar: { type: "integer" } bar: { type: "integer" }
, ( foo, bar ) -> }, function(foo, bar) {
console.log "arguments are valid" return console.log("arguments are valid");
});
foo(); // fail please! foo(); // fail please!
TYPESHAVE
uses the established jsonschema validation-format, which allows extensive and nested datavalidation.
See the npm module here
IN THE BROWSER
<script src="https://raw.githubusercontent.com/coderofsalvation/typeshave.js/master/browser/typeshave.min.js"></script>
<script>
typeshave = require("typeshave").typesafe;
var foo = typeshave({
foo: { type: "string" },
bar: { type: "boolean" }
}, function(foo,bar){
alert("ok data passed!");
});
foo( "string", true );
</script>
Why non-typesafe is great, except with PHAT nested container-objects
For example:
- REST payloads
- objects which represent configs or options
- datastructures and resultsets for html-rendering or processing purposes
Are you still passing phat data around fingers-crossed
-style?
Still wondering why functions like this explode once in a while? :D
JS: foo( { foo:"bar", bar: 123, records: [ 1, 2 ] } );
PHP: foo( (object)array( "foo"=>"bar", "bar"=>123, "records": array( 1, 2 )) );
Did you you try fixing your code with if/else checks? well good luck..
JS: if( data == undefined data.bar == undefined || bar == undefined || Argh this is a big PITA
JS: // omg how do I even check properties recursively?
JS: // now finally we can do what the function should do :/
and in PHP
PHP: function foo($data){
PHP: if( isset($data) &&
PHP: is_object($data) &&
PHP: isset($data->foo) &&
PHP: is_string($data->foo) &&
PHP: ..
PHP: &&
PHP: ..
PHP: && Argh this is a big PITA
PHP: // omg how do I even check properties recursively?
PHP: foreach( $data->records as $record ){
PHP: // PITA
PHP: // PITA
PHP: // PITA
PHP: }
PHP: ...
PHP: // now finally we can do what the function should do :/
PHP: }
TYPESHAVE
and a simple jsonschema like this would validate the calls above easily:
{
"type": "object",
"properties": {
"foo": { "type": "string", "enum": ["bar","foo"] },
"bar": { "type": "integer", minimum:1, maximum:100 },
"records": {
"type": "array",
"items":[{
"type":"integer"
}]
}
}
}
More than typesafe
Jsonschema can do more than nested typechecking, check the docs here
{
foo:{
type: "string",
enum: ["simple","advanced","custom"]
},
bar:{
type: "string",
minLength: 1,
maxLength: 100,
regex: "/[A-Za-z]/"
}
}
Conclusion
With typeshave you can replace all that with one line of code. No more :
- functions going out of control
- assertions-bloat inside functions
- complaining about javascript not being typesafe
- typesafe nested datastructures
- verbose unittests doing typesafe stuff
Typeshave deals with problems immediately when they occur to prevent this:
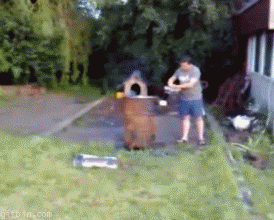